It turned out to be harder that I expected to setup a Jabber (XMPP) server on an Ubuntu virtual machine (
ami-ce44a1a7) within
Amazon EC2. I chose to setup
ejabberd since it was an easy to install via apt-get. I was a little nervous about working with a server built with
Erlang since I knew nothing about it, but it was a reputable server and I was counting on not needing to have any Erlang knowledge to work with ejabberd. That was a correct assumption for the most part.
First, I logged into the VM and installed ejabberd:
apt-get install ejabberd
Next I edited the ejabberd config file,
/etc/ejabberd/ejabberd.cfg
, making
kweiner@jabber.pop140.com
an admin user and setting the hostname as
jabber.pop140.com
:
%% Admin user
{acl, admin, {user, "kweiner", "jabber.pop140.com"}}.
%% Hostname
{hosts, ["jabber.pop140.com"]}.
Next, I restarted the server and registered kweiner as a new user:
/etc/init.d/ejabberd rest
art
ejabberdctl register kweiner jabber.pop140.com mypasswd
Then I authorized traffic on ports 5222 (Jabber), 5223 (Jabber encrypted for old clients), 5269 (Other Jabber servers), and 5280 (Jabber web admin tool):
ec2-authorize default -p 5222
ec2-authorize default -p 5223
ec2-authorize default -p 5269
ec2-authorize default -p 5280
I could now hit the admin screen on which I logged in as
kweiner@jabber.pop140.com
:
http://jabber.pop140.com:5280/admin/
From here it was possible to add new users and browse server statistics. I experimented with adding users and using various Jabber clients like
Gajim to send messages from one user to another.
My first problem came when I tried to communicate with users registered in other Jabber servers like
Jabber.org and
Google Talk. I struggled for hours trying to figure out why users on my server couldn't communicate with users from these other servers. Thankfully
James Murty gave me a bit of help on this
Jabber on EC2 message board thread. It turned out that I needed to configure
SRV records in my DNS settings.
I logged into GoDaddy where my domain is registered and configured SRV records as follows:
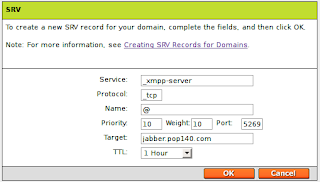
After this, I was able to use nslookup to verify that the SRV records were setup properly:
kweiner~$ nslookup
> set type=srv
> _xmpp-server._tcp.pop140.com
Server: 66.75.160.63
Address: 66.75.160.63#53
Non-authoritative answer:
_xmpp-server._tcp.pop140.com service = 10 10 5269 jabber.pop140.com.
That did it! My jabber server was finally federating with other jabber servers and my users could talk to their users.
I encountered my next big problem when I tried to use jabber again after terminating and relaunching my AMI. ejabberd failed to start and I found the following error message in the
/var/log/ejabberd/ejabberd.log
:
application: ejabberd
exited: {bad_return,{{ejabberd_app,start,[normal,[]]},
{'EXIT',{{badmatch,{aborted,{no_exists,config}}},
[{ejabberd_config,set_opts,1},
{ejabberd_app,start,2},
{application_master,start_it_old,4}]}}}}
After some googling, I found that ejabberd associates itself with an Erlang node name, a concept I don't really understand that well. By default the node name was dynamically set based on the hostname for the machine. It looked something like this:
ejabberd@domU-12-31-38-00-9D-63
. This node name is somehow linked to the
Mnesia database stored as files within
/var/lib/ejabberd
. The problem is that the hostname and therefore the node name changes everytime the AMI is relaunched which confuses ejabberd.
One solution I found is to explicitly set the node name. I did this by modifying
/etc/default/ejabberd
adding the line:
export ERLANG_NODE=ejabberd@jabber
This requires adding
jabber
as a host name inside
/etc/hosts
:
127.0.0.1 localhost.localdomain localhost jabber
I made those changes, removed all the database files from
/var/lib/ejabberd
, and restarted ejabberd. That did it! The node name was the same regardless of the hostname associated with the particular AMI instance.
This was a lot of effort, but it probably would have been easier if I had been familiar with Erlang applications and SRV DNS settings. I hope this post helps someone else struggling to setup ejabberd on EC2 as I did.